OpenPyXLを使って、下線を設定します。
import openpyxl
# ワークブックを開く
wb = openpyxl.Workbook()
# アクティブシートを取得
sh = wb.active
# セルの取得
cell1 = sh.cell(row=1,column=1)
cell2 = sh.cell(row=2,column=1)
cell3 = sh.cell(row=3,column=1)
cell4 = sh.cell(row=4,column=1)
cell5 = sh.cell(row=5,column=1)
# セルに値を入れ込む
cell1.value = "AAA"
cell2.value = "BBB"
cell3.value = "CCC"
cell4.value = "DDD"
cell5.value = "EEE"
# 下線の有無 確認
underline1 = cell1.font.underline
print(underline1)
# 下線の設定
cell1.font = openpyxl.styles.Font(underline="single")
cell2.font = openpyxl.styles.Font(underline="double")
cell3.font = openpyxl.styles.Font(underline="singleAccounting")
cell4.font = openpyxl.styles.Font(underline="doubleAccounting")
cell5.font = openpyxl.styles.Font(underline=None)
# 下線の有無 確認
underline2 = cell2.font.underline
print(underline2)
# ワークブック保存
wb.save('file.xlsx')
# ワークブックを閉じる
wb.close()
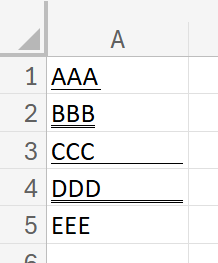
プログラムのコードを最初から見ていきます。
import openpyxl
openpyxlをインポートします。これをしないとopenpyxlのモジュールが使えません
# ワークブックを開く
wb = openpyxl.Workbook()
openpyxlモジュールの、Workbook()関数を使い、新規のエクセルファイルを作成します。
新規のエクセルファイル作成と同時にシート名が”Sheet”が自動で作成されます。
# アクティブシートを取得
sh = wb.active
アクティブのシートを取得します。このエクセルファイルには”Sheet”のみしか入っていないため、このシートのオブジェクトが入ります。
# セルの取得
cell1 = sh.cell(row=1,column=1)
cell2 = sh.cell(row=2,column=1)
cell3 = sh.cell(row=3,column=1)
cell4 = sh.cell(row=4,column=1)
cell5 = sh.cell(row=5,column=1)
セルを取得します。sh.cell(row=1,column=1)で、rowが行、columnが列、1行目/1列目のセルのオブジェクトを取得します。1列目の1行~5行目のセルのオブジェクトを取得します。
# セルに値を入れ込む
cell1.value = "AAA"
cell2.value = "BBB"
cell3.value = "CCC"
cell4.value = "DDD"
cell5.value = "EEE"
cell.valueとして”AAA”という値をセルに入れ込みます。1行/1列目のセルにAAAという値を入れ込んだ事になります。1列目の1行~5行目のセルに値を入れ込みます。
# 下線の有無 確認
underline1 = cell1.font.underline
print(underline1)
cell1.font.underlineで下線の種類を調べる事ができます。今は何も下線を設定していないため、print(underline1)の出力結果はNoneになります。
# 下線の設定
cell1.font = openpyxl.styles.Font(underline="single")
cell2.font = openpyxl.styles.Font(underline="double")
cell3.font = openpyxl.styles.Font(underline="singleAccounting")
cell4.font = openpyxl.styles.Font(underline="doubleAccounting")
cell5.font = openpyxl.styles.Font(underline=None)
openpyxl.styles.Font( )で下線の設定を行います。underline=” “で下線の種類を指定します。
下線の種類は下記の表の通りです。
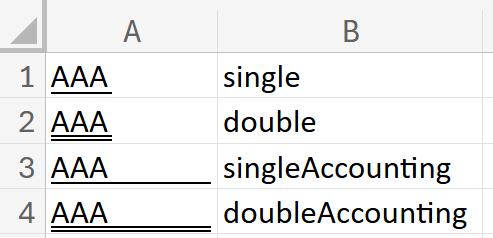
# 下線の有無 確認
underline2 = cell2.font.underline
print(underline2)
cell2.font.underlineで下線の種類を確認でき、print(underline2)で出力すると、doubleとなります。手前でdoubleで指定しているためです。
以上となります。
OpenPyXLの他の関数の使い方について知りたい場合は下記記事が非常に参考になります。